Configuration and Build¶
Initialization of the Stack¶
During initialization, the PUSopen® stack resets all internal services (Section 2.2), their buffers, and observable parameters (Section 4.8) - mostly by filling the memory areas with 0. The initialization is a required step.
The user application initializes the PUSopen® stack by calling API po_init(). po_init() resets the stack’s internal modules and sets observable parameters to their default values.
#include <pusopen/pusopen.h>
int main () {
/* Initialization of the PUSopen */
po_init();
/* Configuration of the PUSopen */
...
/* The rest of the processing starts here */
...
}
Configuration Overview¶
PUSopen® configuration consists of:
macros in the header file poconfig.h (see Section 3.1)
definitions of PUS data items (Section 3.4) and FESS instances added at run-time by the user application code (see Section TBD for APIs and code examples).
customer-specific PUS packet format definitions (see Section TBD).
The configuration file poconfig.h is part of the customer application code. The macros of poconfig.h (Section 3.1) are used during the instantiation of the PUSopen configuration structure po_config_t and instantiation of FESS layers.
The PUSopen® configuration structure po_config_t can be found in the header file pusopen/pocf.h. The template of the poconfig.h file is supplied with the PUSopen®.
Configuration Parameters¶
PUSopen® allows users to configure the internal buffers’ size, total number of FESS instances, and other functionality. This section lists all configurable parameters. The next section 3.2 explains how the configurable parameters are organized in the PUSopen® configuration.
Table PUSopen® Configuration Parameters lists all PUSopen® configuration parameters. Services with no configurable parameters are marked with “n/a”.
Service |
C macro |
Description |
---|---|---|
PUS 1 |
PUS1_RECV_BUF_SIZE |
The size (in bytes) of the PUS 1 reception buffer. The size shall correspond to the size of the largest TM or TC receivable by the PUS 1 provider. |
PUS 3 |
PUS3_REPORT_BUF_SIZE |
The size (in bytes) of the PUS 3 buffer used for assembling TM[3,25] reports. |
PUS 3 |
PUS3_TOTAL_USER_HK |
The total number of user HKs. It may be higher than the initial assumed number of HKs. HKs are added into this statically allocated space at run-time. |
PUS 3 |
PUS3_TOTAL_REPORTS |
The total number of HK reports. Reports are added into this statically allocated space at run-time (e.g., from a configuration file). |
PUS 5 |
PUS5_TOTAL_EVENTS |
The total number of TM[5,x] onboard events (see ECSS PUS standard). The onboard events are added to this statically allocated space at runtime (e.g., from a configuration file). |
PUS 8 |
PUS8_TOTAL_FUNCTIONS |
The total number of custom functions (triggered by PUS 8 TC). The user application code can add custom function definitions to this statically allocated space at runtime. |
PUS 13 |
PUS13_UP_PKT_PAY_SIZE |
The size (in bytes) of the custom payload of the PUS 13 uplink TCs (TC[13,9], TC[13,10], and TC[13,11]). |
PUS 13 |
PUS13_DOWN_PKT_PAY_SIZE |
The size (in bytes) of the custom payload of the PUS 13 downlink TMs (TM[13,1], TM[13,2], and TM[13,3]). |
PUS 17 |
n/a |
|
PUS 20 |
PUS20_REPORT_BUF_SIZE |
The size (in bytes) of the PUS 20 buffer used for assembling TM[20,x] reports. |
PUS User |
PUSUSR_SEND_BUF_SIZE |
The size (in bytes) of the PUS User send buffer. The buffer is used to assemble one TC or TM produced by the PUS User. |
PS |
PS_SEND_BUF_SIZE |
The size (in bytes) of the Packet Service’s send buffer. This buffer is used to assemble one CCSDS Space Packet. |
PS |
PS_RECV_BUF_SIZE |
The size (in bytes) of the Packet Service’s receive buffer. This buffer is used to decode one CCSDS Space Packet. |
PS |
PS_CHECKSUM_TYPE |
The type of checksum added at the end of CCSDS Space Packet’s custom payload (PKT_NOCHECKSUM, PKT_ISO16, or PKT_CRC16). |
FESS |
FESS_TOTAL_INSTANCES |
The total number of the FESS instances in the current integration of the PUSopen®. The user can allocate space for more FESS instances than used. Each FESS instance is statically allocated in the user code and assigned to this statically allocated array at run-time. |
Routing |
PO_ROUTING_TABLE_SIZE |
The total number of the routing records in the PUSopen® packet routing table. The routing records are added to this statically allocated routing table at run-time (e.g., from a configuration file). |
Virtual Channels |
VCS_TOTAL_VIRTUAL_CHANNELS |
The total number of Virtual Channels (0-64). This total may be higher than the actual number of virtual channels used. Each Virtual Channel is statically instantiated in the user application code and added to this static array of Virtual Channels at run-time. |
VCS_MCID |
The MCID used by the Virtual Channel Services. |
|
VCS_TFVN |
The TFVN used by the Virtual Channel Services. |
|
VCS_SEND_FRM_BUF_SIZE |
The size (in bytes) of the send buffer used to create a single CCSDS Transfer Frame. |
|
VCS_RECV_FRM_BUF_SIZE |
The size (in bytes) of the receive buffer used to create a single CCSDS Transfer Frame. |
|
VCS_SEND_FRM_TYPE |
Type of the CCSDS Transfer Frame Header used while creating a Transfer Frame (FRAME_USLP_FULL, FRAME_USLP_TRUNCATED). |
|
VCS_RECV_FRM_TYPE |
Type of the CCSDS Transfer Frame Header used while decoding a received Transfer Frame (FRAME_USLP_FULL, FRAME_USLP_TRUNCATED). |
Note
We encourage setting the buffer size with a margin. The actual operations may require the creation or reception of larger packets than initially designed. The configured size of PUSopen® buffers and the total number of instances (HKs, Reports, Event, FESS, …) shall reflect the mission lifetime of the PUS and CCSDS usage (i.e., configure it a bit higher to allow for expansion during the mission).
PUS Data Items¶
This section lists the members of the C structures representing the PUS5 onboard events, PUS HK, PUS HK reports, and the PUS user-defined functions. Definitions of these PUS data items are added at runtime by the user-application code (e.g., from a user-defined configuration file) into the statically allocated arrays (see Section 3.1).
See Appendix A for APIs used to define the PUS data items described below. The C structures of the PUS data items can be found in the header file pusopen/potypes.h.
PUS on-board event:
C structure member |
Description |
---|---|
id |
Unique PUS onboard event ID. |
level |
Criticality level of the PUS event (values: PUS_EVT_INFO, PUS_EVT_LOW, PUS_EVT_MEDIUM, PUS_EVT_HIGH). |
dataLen |
Length (in bytes) of the data associated with the event. |
PUS user-defined function:
C structure member |
Description |
---|---|
id |
Unique PUS user function ID. |
addr |
The address of the function. This is the C function represented by the function name. |
PUS Housekeeping Parameter (HK):
C structure member |
Description |
---|---|
id |
Unique HK ID. |
type |
The data type of the HK (values: PO_UINT8, PO_UINT16, PO_UINT32, PO_INT8, PO_INT16, PO_INT32, PO_FLOAT32). |
addr |
Address of the HK in memory, either represented by the hex-formatted number or C variable name. |
PUS Housekeeping Report (HK report):
C structure member |
Description |
---|---|
id |
Unique HK Report ID. |
enabled |
The flag that generation of this report is enabled. |
interval |
Period with which the report will be produced by PUS 3. |
sinceLast |
The last time when the report was generated. |
destApuid |
Destination APUID of this HK report. |
obparams |
List of HK parameters included in the report. |
numHk |
A total number of HKs included in the report. |
Callback Functions¶
PUSopen® uses callback functions to notify the user application code about the reception of a certain TM/TC or the need to provide certain data (e.g., current time). All PUSopen® callback functions are declared in a single header file, “pusopen/pocallbacks.h.”
The user can define a custom body for a specific PUSopen® callback or not and use its setter API to make the stack use it. All PUSopen® callbacks have an internal default implementation, so if the user doesn’t set the new instance of a callback, the default variant is used. Here is the list of callbacks:
C callback (ptr) |
Setter function |
Description |
---|---|---|
po_fn_tm_t |
po_set_callback_tm |
Notification of PUS TM reception |
po_fn_tc_t |
po_set_callback_tc |
Notification of PUS TC reception |
po_fn_time_t |
po_set_callback_time |
Request to provide current time |
po_fn_pkt_seq_t |
po_set_callback_pkt_seq |
Request to provide CCSDS packet counter |
po_fn_tm_seq_t |
po_set_callback_tm_seq |
Request to provide PUS TM packet counter |
po_fn_addr_t |
(see Section 5.7) |
PUS 8 custom function triggered by TC[8,1] |
po_fn_subnet_request_t |
po_set_callback_subnet_request |
Subnetwork request implementation |
po_fn_subnet_indication_t |
po_set_callback_subnet_indication |
Subnetwork indication implementation |
po_set_callback_debug (see Section 4.9) |
Custom callback for PUSopen® debug log |
Build Process¶
PUSopen® user applications are composed of the supplied PUSopen® header files, PUSopen® custom-defined configuration, and the PUSopen® library (.a, .so, or .dll). All these source components are compiled and linked into a single executable (ur custom library).
PUSopen® doesn’t have any external dependencies (neither in the Operating System nor in the user code). The only part that is required from the user application code is to instantiate the PUSopen® configuration (C structure po_config_t, Section 3.2), define selected PUSopen® callback functions (Section 3.5), and call the PUSopen® APIs sending and receiving the CCSDS and PUS packets.
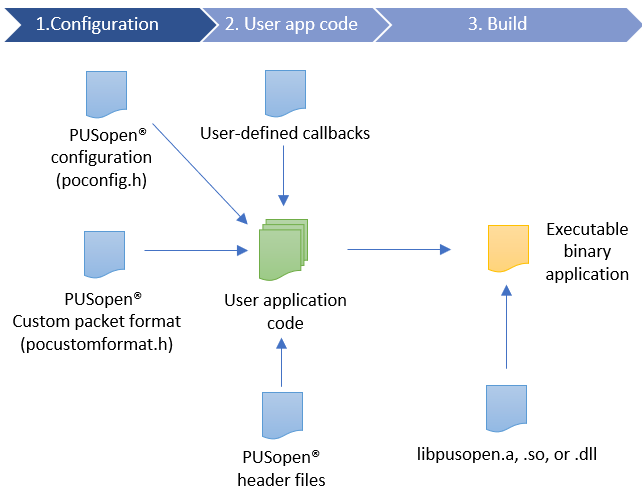
Fig. 10 PUSopen® User Application Build Process¶
Before the build, please use the supplied “setenv” scripts to set the environment variables. Alternatively, review the “setenv” scripts and set the same environment variables by your own means (GCC compiler, linker, C flags, etc.).
During the linking process the PUSopen® static library (.a) or dynamic library (.so, or .dll) is linked with the rest of the user application code. The following example shows how to link the PUSopen® library with the customer application (GCC compiler/linker):
gcc -L../pusopen/lib/_WIN32 -o po.exe main.o -lws2_32 -lpusopen
The supplied README.md file described the whole build process in detail.
Header files¶
PUSopen® stack is distributed with a set of header files that contain declarations of the APIs, structures, and types. User application code shall only include a single header file: pusopen/pusopen.h. This header file includes all other necessary header files of the PUSopen® stack, making them available to the user application code.
User application code shall not include any other PUSopen® header file than “pusopen/pusopen.h”.
Build Flags¶
PUSopen® build scripts derive the build flags according to the setting of the specific environment variables. See the supplied “setenv” scripts for the list of environment variables.